PINE LIBRARY
已更新 HighTimeframeSampling
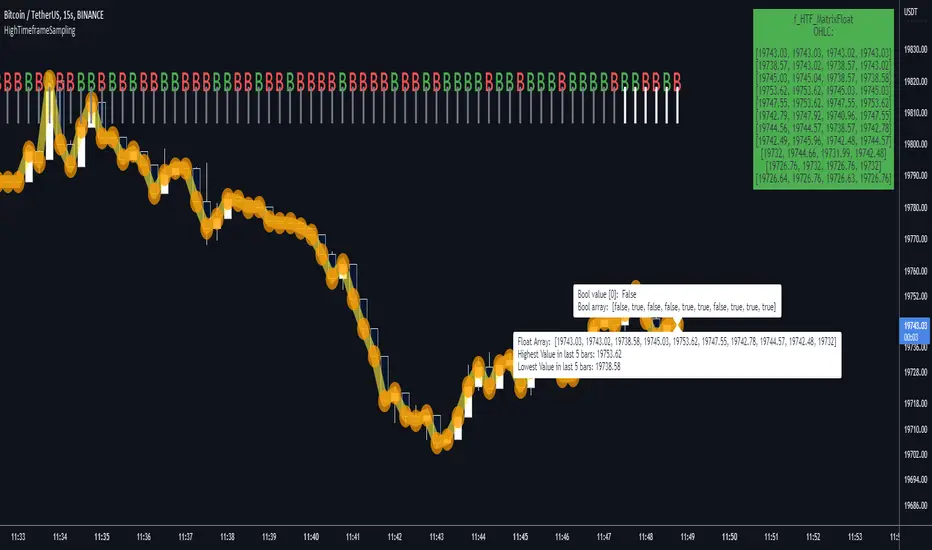
Library "HighTimeframeSampling"
Library for sampling high timeframe (HTF) data. Returns an array of historical values, an arbitrary historical value, or the highest/lowest value in a range, spending a single security() call.
An optional pass-through for the chart timeframe is included. Other than that case, the data is fixed and does not alter over the course of the HTF bar. It behaves consistently on historical and elapsed realtime bars.
The first version returns floating-point numbers only. I might extend it if there's interest.
🙏 Credits: This library is (yet another) attempt at a solution of the problems in using HTF data that were laid out by Pinecoders - to whom, especially to Luc F, many thanks are due - in "security() revisited" - tradingview.com/script/00jFIl5w-security-revisited-PineCoders/, which I recommend you consult first. Go ahead, I'll wait.
All code is my own.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
WHAT'S THE PROBLEM? OR, WHY NOT JUST USE SECURITY()
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
There are many difficulties with using HTF data, and many potential solutions. It's not really possible to convey it only in words: you need to see it on a chart.
Before using this library, please refer to my other HTF library, HighTimeframeTiming: tradingview.com/script/F9vFt8aX-HighTimeframeTiming/, which explains it extensively, compares many different solutions, and demonstrates (what I think are) the advantages of using this very library, namely, that it's stable, accurate, versatile and inexpensive. Then if you agree, come back here and choose your function.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
MOAR EXPLANATION
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
🧹 Housekeeping: To see which plot is which, turn line labels on: Settings > Scales > Indicator Name Label. Vertical lines at the top of the chart show the open of a HTF bar: grey for historical and white for real-time bars.
‼ LIMITATIONS: To avoid strange behaviour, use this library on liquid assets and at chart timeframes high enough to reliably produce updates at least once per bar period.
A more conventional and universal limitation is that the library does not offer an unlimited view of historical bars. You need to define upfront how many HTF bars you want to store. Very large numbers might conceivably run into data or performance issues.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
BRING ON THE FUNCTIONS
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Function f_HTF_Value(string _HTF, float _source, int _arrayLength, int _HTF_Offset, bool _useLiveDataOnChartTF=false)
Returns a floating-point number from a higher timeframe, with a historical operator within an abitrary (but limited) number of bars.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to store and must be greater than zero. You can't go back further in history than this number of bars (minus one, because the current/most recent available bar is also stored).
param int _HTF_Offset is the historical operator for the value you want to return. E.g., if you want the most recent fixed close, _source=close and _HTF_Offset = 0. If you want the one before that, _HTF_Offset=1, etc.
The number of HTF bars to look back must be zero or more, and must be one less than the number of bars stored.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches the raw source values from security(){0}.
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
Returns a floating-point value that you requested from the higher timeframe.
Function f_HTF_Array(string _HTF, float _source, int _arrayLength, bool _useLiveDataOnChartTF=false, int _startIn, int _endIn)
Returns an array of historical values from a higher timeframe, starting with the current bar. Optionally, returns a slice of the array. The array is in reverse chronological order, i.e., index 0 contains the most recent value.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to keep in the array.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
param int _startIn is the array index to begin taking a slice. Must be at least one less than the length of the array; if out of bounds it is corrected to 0.
param int _endIn is the array index BEFORE WHICH to end the slice. If the ending index of the array slice would take the slice past the end of the array, it is corrected to the end of the array. The ending index of the array slice must be greater than or equal to the starting index. If the end is less than the start, the whole array is returned. If the starting index is the same as the ending index, an empty array is returned. If either the starting or ending index is negative, the entire array is returned (which is the default behaviour; this is effectively a switch to bypass the slicing without taking up an extra parameter).
Returns an array of HTF values.
Function f_HTF_Highest(string _HTF="", float _source, int _arrayLength, bool _useLiveDataOnChartTF=true, int _rangeIn)
Returns the highest value within a range consisting of a given number of bars back from the most recent bar.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to store and must be greater than zero. You can't have a range greater than this number.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
param _rangeIn is the number of bars to include in the range of bars from which we want to find the highest value. It is NOT the historical operator of the last bar in the range. The range always starts at the current bar. A value of 1 doesn't make much sense but the function will generously return the only value it can anyway. A value less than 1 doesn't make sense and will return an error. A value that is higher than the number of stored values will be corrected to equal the number of stored values.
Returns a floating-point number representing the highest value in the range.
Function f_HTF_Lowest(string _HTF="", float _source, int _arrayLength, bool _useLiveDataOnChartTF=true, int _rangeIn)
Returns the lowest value within a range consisting of a given number of bars back from the most recent bar.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to store and must be greater than zero. You can't go back further in history than this number of bars (minus one, because the current/most recent available bar is also stored).
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
param _rangeIn is the number of bars to include in the range of bars from which we want to find the highest value. It is NOT the historical operator of the last bar in the range. The range always starts at the current bar. A value of 1 doesn't make much sense but the function will generously return the only value it can anyway. A value less than 1 doesn't make sense and will return an error. A value that is higher than the number of stored values will be corrected to equal the number of stored values.
Returns a floating-point number representing the lowest value in the range.
Library for sampling high timeframe (HTF) data. Returns an array of historical values, an arbitrary historical value, or the highest/lowest value in a range, spending a single security() call.
An optional pass-through for the chart timeframe is included. Other than that case, the data is fixed and does not alter over the course of the HTF bar. It behaves consistently on historical and elapsed realtime bars.
The first version returns floating-point numbers only. I might extend it if there's interest.
🙏 Credits: This library is (yet another) attempt at a solution of the problems in using HTF data that were laid out by Pinecoders - to whom, especially to Luc F, many thanks are due - in "security() revisited" - tradingview.com/script/00jFIl5w-security-revisited-PineCoders/, which I recommend you consult first. Go ahead, I'll wait.
All code is my own.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
WHAT'S THE PROBLEM? OR, WHY NOT JUST USE SECURITY()
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
There are many difficulties with using HTF data, and many potential solutions. It's not really possible to convey it only in words: you need to see it on a chart.
Before using this library, please refer to my other HTF library, HighTimeframeTiming: tradingview.com/script/F9vFt8aX-HighTimeframeTiming/, which explains it extensively, compares many different solutions, and demonstrates (what I think are) the advantages of using this very library, namely, that it's stable, accurate, versatile and inexpensive. Then if you agree, come back here and choose your function.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
MOAR EXPLANATION
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
🧹 Housekeeping: To see which plot is which, turn line labels on: Settings > Scales > Indicator Name Label. Vertical lines at the top of the chart show the open of a HTF bar: grey for historical and white for real-time bars.
‼ LIMITATIONS: To avoid strange behaviour, use this library on liquid assets and at chart timeframes high enough to reliably produce updates at least once per bar period.
A more conventional and universal limitation is that the library does not offer an unlimited view of historical bars. You need to define upfront how many HTF bars you want to store. Very large numbers might conceivably run into data or performance issues.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
BRING ON THE FUNCTIONS
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Function f_HTF_Value(string _HTF, float _source, int _arrayLength, int _HTF_Offset, bool _useLiveDataOnChartTF=false)
Returns a floating-point number from a higher timeframe, with a historical operator within an abitrary (but limited) number of bars.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to store and must be greater than zero. You can't go back further in history than this number of bars (minus one, because the current/most recent available bar is also stored).
param int _HTF_Offset is the historical operator for the value you want to return. E.g., if you want the most recent fixed close, _source=close and _HTF_Offset = 0. If you want the one before that, _HTF_Offset=1, etc.
The number of HTF bars to look back must be zero or more, and must be one less than the number of bars stored.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches the raw source values from security(){0}.
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
Returns a floating-point value that you requested from the higher timeframe.
Function f_HTF_Array(string _HTF, float _source, int _arrayLength, bool _useLiveDataOnChartTF=false, int _startIn, int _endIn)
Returns an array of historical values from a higher timeframe, starting with the current bar. Optionally, returns a slice of the array. The array is in reverse chronological order, i.e., index 0 contains the most recent value.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to keep in the array.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
param int _startIn is the array index to begin taking a slice. Must be at least one less than the length of the array; if out of bounds it is corrected to 0.
param int _endIn is the array index BEFORE WHICH to end the slice. If the ending index of the array slice would take the slice past the end of the array, it is corrected to the end of the array. The ending index of the array slice must be greater than or equal to the starting index. If the end is less than the start, the whole array is returned. If the starting index is the same as the ending index, an empty array is returned. If either the starting or ending index is negative, the entire array is returned (which is the default behaviour; this is effectively a switch to bypass the slicing without taking up an extra parameter).
Returns an array of HTF values.
Function f_HTF_Highest(string _HTF="", float _source, int _arrayLength, bool _useLiveDataOnChartTF=true, int _rangeIn)
Returns the highest value within a range consisting of a given number of bars back from the most recent bar.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to store and must be greater than zero. You can't have a range greater than this number.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
param _rangeIn is the number of bars to include in the range of bars from which we want to find the highest value. It is NOT the historical operator of the last bar in the range. The range always starts at the current bar. A value of 1 doesn't make much sense but the function will generously return the only value it can anyway. A value less than 1 doesn't make sense and will return an error. A value that is higher than the number of stored values will be corrected to equal the number of stored values.
Returns a floating-point number representing the highest value in the range.
Function f_HTF_Lowest(string _HTF="", float _source, int _arrayLength, bool _useLiveDataOnChartTF=true, int _rangeIn)
Returns the lowest value within a range consisting of a given number of bars back from the most recent bar.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float _source is the source value that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _arrayLength is the number of HTF bars you want to store and must be greater than zero. You can't go back further in history than this number of bars (minus one, because the current/most recent available bar is also stored).
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
param _rangeIn is the number of bars to include in the range of bars from which we want to find the highest value. It is NOT the historical operator of the last bar in the range. The range always starts at the current bar. A value of 1 doesn't make much sense but the function will generously return the only value it can anyway. A value less than 1 doesn't make sense and will return an error. A value that is higher than the number of stored values will be corrected to equal the number of stored values.
Returns a floating-point number representing the lowest value in the range.
發行說明
v2New function: f_HTF_MatrixFloat()
Function f_HTF_MatrixFloat(string _HTF="", float[] _sourceArray, int _matrixHistory, bool _useLiveDataOnChartTF=true)
Returns a matrix of historical values *of an array* from a higher timeframe, starting with the current bar. Unlike f_HTF_ArrayFloat, there is no option to return a slice of the matrix. The matrix is in reverse chronological order, i.e., row 0 contains the most recent values.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param float[] _sourceArray is the source array that you want to sample, e.g. close, open, etc., or you can use any floating-point number.
param int _matrixHistory is the number of HTF bars for which you want to keep arrays in the matrix.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
Returns a matrix of historical HTF array values.
Other changes
Renamed all existing functions to include "Float" in the title: future-proofing in case I extend this library to bools or what-have you.
- f_HTF_Value() becomes f_HTF_Float()
- f_HTF_Array() becomes f_HTF_ArrayFloat()
- f_HTF_Highest() becomes f_HTF_HighestFloat()
- f_HTF_Lowest() becomes f_HTF_LowestFloat()
Renamed input _arrayLength to _arrayHistory for all existing functions that use arrays.
Added a couple more limitations to the list.
Updated the section that gets raw HTF data to use a helper function to get multiple values that we can use as an array.
Fixed some minor naming and logic issues in the functions.
Added:
f_HTF_Float()
f_HTF_ArrayFloat()
f_HTF_HighestFloat()
f_HTF_LowestFloat()
f_HTF_MatrixFloat()
Removed:
f_HTF_Value()
f_HTF_Array()
f_HTF_Highest()
f_HTF_Lowest()
發行說明
v3Changed all instances of ta.change(time) to timeframe.change().
發行說明
v4Added two new functions to return boolean values.
Corrected some errors in the text.
Made more variable names specific to the type of variable returned.
Fixed the label for the array slice in float function 2.
New functions:
Function f_HTF_Bool(string _HTF, bool _source, int _arrayHistory, int _HTF_Offset, bool _useLiveDataOnChartTF=false)
Returns a boolean value from a higher timeframe, with a historical operator within an abitrary (but limited) number of bars.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param bool _source is the source value that you want to sample.
param int _arrayHistory is the number of HTF bars you want to store and must be greater than zero. You can't go back further in history than this number of bars (minus one, because the current/most recent available bar is also stored).
param int _HTF_Offset is the historical operator for the value you want to return. E.g., if you want the most recent fixed close, _source=close and _HTF_Offset = 0. If you want the one before that, _HTF_Offset=1, etc.
The number of HTF bars to look back must be zero or more, and must be one less than the number of bars stored.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches the raw source values from security()[0].
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
Returns a boolean value that you requested from the higher timeframe.
Function f_HTF_ArrayBool(string _HTF, bool _source, int _arrayHistory, bool _useLiveDataOnChartTF=false, int _startIn, int _endIn)
Returns an array of historical values *of a single variable* from a higher timeframe, starting with the current bar. Optionally, returns a slice of the array. The array is in reverse chronological order, i.e., index 0 contains the most recent value.
param string _HTF is the string that represents the higher timeframe. It must be in a format that the request.security() function recognises. The input timeframe cannot be lower than the chart timeframe or an error is thrown.
param bool _source is the source value that you want to sample.
param int _arrayHistory is the number of HTF bars you want to keep in the array.
param bool _useLiveDataOnChartTF uses live data on the chart timeframe.
If the higher timeframe is the same as the chart timeframe, store the live value (i.e., from this very bar). For all truly higher timeframes, store the fixed value (i.e., from the previous bar).
The default is to use live data for the chart timeframe, so that this function works intuitively, that is, it does not fix data unless it has to (i.e., because the data is from a higher timeframe).
This means that on default settings, on the chart timeframe, it matches raw source values from security().
You can override this behaviour by passing _useLiveDataOnChartTF as false. Then it will fix all data for all timeframes.
param int _startIn is the array index to begin taking a slice. Must be at least one less than the length of the array; if out of bounds it is corrected to 0.
param int _endIn is the array index BEFORE WHICH to end the slice. If the ending index of the array slice would take the slice past the end of the array, it is corrected to the end of the array. The ending index of the array slice must be greater than or equal to the starting index. If the end is less than the start, the whole array is returned. If the starting index is the same as the ending index, an empty array is returned. If either the starting or ending index is negative, the entire array is returned (which is the default behaviour; this is effectively a switch to bypass the slicing without taking up an extra parameter).
Returns an array of HTF values.
Added the raw HTF data to support them, and examples of usage.
Pine腳本庫
秉持 TradingView 一貫的共享精神,作者將此 Pine 程式碼發佈為開源庫,讓社群中的其他 Pine 程式設計師能夠重複使用。向作者致敬!您可以在私人專案或其他開源發佈中使用此庫,但在公開發佈中重複使用該程式碼需遵守社群規範。
🆓 All my free scripts: simplecrypto.life/free-scripts/
💰 Trade with me: simplecrypto.life/trade-with-me/
🙋 Free help with Pinescript: simplecrypto.life/get-help-with-pinescript/
💰 Trade with me: simplecrypto.life/trade-with-me/
🙋 Free help with Pinescript: simplecrypto.life/get-help-with-pinescript/
免責聲明
這些資訊和出版物並不意味著也不構成TradingView提供或認可的金融、投資、交易或其他類型的意見或建議。請在使用條款閱讀更多資訊。
Pine腳本庫
秉持 TradingView 一貫的共享精神,作者將此 Pine 程式碼發佈為開源庫,讓社群中的其他 Pine 程式設計師能夠重複使用。向作者致敬!您可以在私人專案或其他開源發佈中使用此庫,但在公開發佈中重複使用該程式碼需遵守社群規範。
🆓 All my free scripts: simplecrypto.life/free-scripts/
💰 Trade with me: simplecrypto.life/trade-with-me/
🙋 Free help with Pinescript: simplecrypto.life/get-help-with-pinescript/
💰 Trade with me: simplecrypto.life/trade-with-me/
🙋 Free help with Pinescript: simplecrypto.life/get-help-with-pinescript/
免責聲明
這些資訊和出版物並不意味著也不構成TradingView提供或認可的金融、投資、交易或其他類型的意見或建議。請在使用條款閱讀更多資訊。